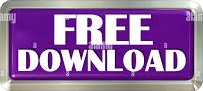
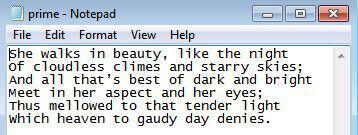
In Python, the os.path submodule contains functions exclusively designed to operate with file paths. Note: A path is the unique location of a file or directory in a filesystem It returns a boolean based on the existence of the path. exists() function which accepts the path to the file or directory as an argument. To check if a file or folder exists we can use the path. The os module provides multiple functions to interact with the operative system. Let’s dive into other options to achieve this. This method is extremely useful when writing in files, but results inefficient if we only want to check if a file exists. It allocates and releases resources precisely, therefore we won’t need to close the file. If we don’t want to close the file by ourselves, we could use the with context manager. It’s considered a good practice according to the Python documentation.Ĭalling file.write() without using the with keyword or calling file.close() might result in the arguments of file.write() not being completely written to the disk, even if the program exits successfully.Įven if we’re not writing to the file, it’s extremely recommended to close the file because it could lead to multiple performance problems. Note how we’re closing the file right after we opened it. : print('Sorry the file we\'re looking for doesn\'t exist') Let’s see what happens when the file we’re looking for actually exists. Note how the exit() function will only execute if an exception is raised.
PYTHON FIND FILE CODE
In the code above we’re printing a custom message and stopping the execution of the program if the file doesn’t exist. Sorry the file we're looking for doesn't exist : print('Sorry the file we\'re looking for doesn\' exist') We can take advantage of this, and handle the exception in case the file we’re looking for doesn’t exist. In : open('im-not-here.txt')įileNotFoundError: No such file or directory: 'im-not-here.txt' If you try to open a file that doesn’t exist, Python will raise a FileNotFoundError. Now you’re all set let’s dive into ways to check if a folder or file exists in Python.
PYTHON FIND FILE INSTALL
pip install ipythonĪfter doing this, you’ll get access to a beautiful Python shell just by typing ipython. This is just a commodity, therefore not strictly necessary. Note: If you’re using Windows, set up that file simple file structure with a graphical file manager.įinally, we’ll be using Ipython as our interactive Python shell which gives a pretty interface to work with. The files can be empty since we won’t need to read their content, The commands above create a file to play with, a testing directory, and another file inside the testingdirectory. We’ll be using some test files along with this tutorial, so make sure to create the following files: touch testfile.txt Check out our Python installation guide if you don’t have Python 3 installed. If you got a 2.x version, you’ll need to use the “python3” command. Open your terminal and type the following command: python -version Before startingīefore executing any command below, make sure you have Python 3 installed in your system. In today’s tutorial, you’ll learn some quick ways to check if a file or folder exists in Python. Your program could become useless if a specific file isn’t in place at the moment of execution.
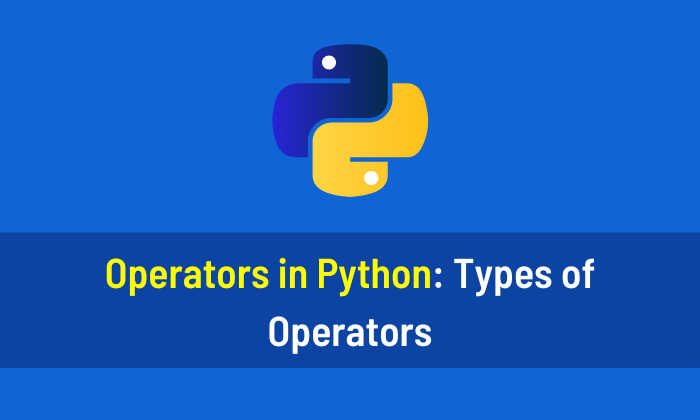
In this tutorial, you’ll learn different ways to check the existence of a file or directory using only built-in modules.Ĭhecking if a file or script is in the correct spot is crucial for any CLI program. The Python standard library contains most of the functionality a developer would need to solve a problem.
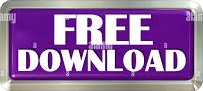